ㆍLinq
- 질의어로 필터링
<예제>
using System;
using System.Linq;
using System.Collections.Generic;
class Class1
{
static void Main(string[] args)
{
List<int> num = new List<int>(Enumerable.Range(0, 100));
var query = from data in num
where data % 2 == 0
select data;
foreach (var i in query)
Console.WriteLine(i);
}
}
<group 절>
group A(범위변수) by B(분류기준) into C(그룹변수)
추가적 쿼리 작업 없는 경우에는 into와 그룹 변수를 쓰지 않아도 괜찮습니다.
public class Student
{
public string Name { get; set; }
public double Score { get; set; }
}
class Ex
{
static void Main(string[] args)
{
List<Student> listStudent = new List<Student>
{
new Student {Name = "철수", Score = 87.5},
new Student {Name = "영희", Score = 77.5},
new Student {Name = "민수", Score = 67.5},
new Student {Name = "짱구", Score = 91.2},
new Student {Name = "맹구", Score = 66.1}
};
var queryStudent = from i in listStudent
orderby i.Score
group i by i.Score < 80.0;
foreach (var studentGroup in queryStudent)
{
Console.WriteLine(studentGroup.Key ? "80점 미만" : "80점 이상");
foreach (var i in studentGroup)
Console.WriteLine($"{i.Name}, {i.Score}");
}
}
}
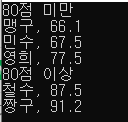
<그룹변수 추가>
using System;
using System.Linq;
using System.Collections.Generic;
public class Student
{
public string Name { get; set; }
public double Score { get; set; }
}
class Ex
{
static void Main(string[] args)
{
List<Student> listStudent = new List<Student>
{
new Student {Name = "철수", Score = 87.5},
new Student {Name = "영희", Score = 77.5},
new Student {Name = "민수", Score = 67.5},
new Student {Name = "짱구", Score = 91.2},
new Student {Name = "맹구", Score = 66.1}
};
var queryStudent = from i in listStudent
group i by (int)i.Score / 10 into g
orderby g.Key
select g;
foreach (var studentGroup in queryStudent)
{
int temp = studentGroup.Key * 10;
Console.WriteLine($"{temp}이상{temp + 10}미만");
foreach (var i in studentGroup)
Console.WriteLine($"\t{i.Name}: {i.Score} 점");
}
}
}
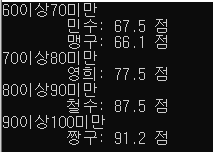
<내부 join>
a-field n b-field
from a in A
join b in B on a-field equals b-field
using System;
using System.Linq;
using System.Collections.Generic;
public class Student
{
public string Name { get; set; }
public double Score { get; set; }
}
public class MyHobby
{
public string Name { get; set; }
public string Hobby { get; set; }
}
class Ex
{
static void Main(string[] args)
{
List<Student> listStudent = new List<Student>
{
new Student {Name = "철수", Score = 87.5},
new Student {Name = "영희", Score = 77.5},
new Student {Name = "민수", Score = 67.5},
new Student {Name = "짱구", Score = 91.2},
new Student {Name = "맹구", Score = 66.1}
};
List<MyHobby> listHobby = new List<MyHobby>
{
new MyHobby {Name = "철수", Hobby = "게임"},
new MyHobby {Name = "영희", Hobby = "당구"},
new MyHobby {Name = "민수", Hobby = "공부"}
};
var queryStudent = from i in listStudent
join j in listHobby on i.Name equals j.Name
select new
{
Name = i.Name,
Score = i.Score,
Hobby = j.Hobby
};
foreach (var studentGroup in queryStudent)
Console.WriteLine($"이름: {studentGroup.Name}, 점수: {studentGroup.Score}, 취미:{studentGroup.Hobby}");
}
}

<외부 join>
a-field u b-field
using System;
using System.Linq;
using System.Collections.Generic;
public class Student
{
public string Name { get; set; }
public double Score { get; set; }
}
public class MyHobby
{
public string Name { get; set; }
public string Hobby { get; set; }
}
class Ex
{
static void Main(string[] args)
{
List<Student> listStudent = new List<Student>
{
new Student {Name = "철수", Score = 87.5},
new Student {Name = "영희", Score = 77.5},
new Student {Name = "민수", Score = 67.5},
new Student {Name = "짱구", Score = 91.2},
new Student {Name = "맹구", Score = 66.1}
};
List<MyHobby> listHobby = new List<MyHobby>
{
new MyHobby {Name = "철수", Hobby = "게임"},
new MyHobby {Name = "영희", Hobby = "당구"},
new MyHobby {Name = "민수", Hobby = "공부"}
};
var queryStudent = from i in listStudent
join j in listHobby on i.Name equals j.Name into g
from j in g.DefaultIfEmpty(new MyHobby {Hobby = "x"})
select new
{
Name = i.Name,
Score = i.Score,
Hobby = j.Hobby
};
foreach (var studentGroup in queryStudent)
Console.WriteLine($"이름: {studentGroup.Name}, 점수: {studentGroup.Score}, 취미:{studentGroup.Hobby}");
}
}
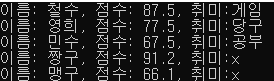
'C# > 공부기록' 카테고리의 다른 글
[C#] Linq / index, value (0) | 2023.01.14 |
---|---|
[C#] 람다식, Func, Action (0) | 2023.01.14 |
[C#] List<T> (0) | 2023.01.14 |
[C#] 어셈블리(assembly) (0) | 2023.01.14 |
[C#]이벤트(event) (0) | 2023.01.14 |